Table of Contents
Introduction
Ever wondered how to measure temperature using Arduino? Whether you’re a beginner or an enthusiast, this guide will walk you through everything step-by-step. We’ll explore different temperature sensors, set up a circuit, write simple code, and troubleshoot common issues.
By the end of this guide, you’ll have a fully functional Arduino-based temperature measurement system!
You may also want to check out how to interface Arduino with a 7-segment display to visualize temperature readings effectively.
What is a Temperature Sensor?
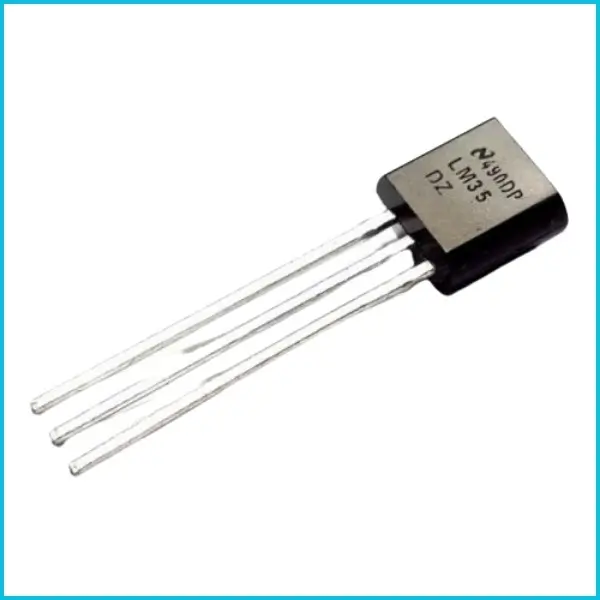
A temperature sensor detects heat and converts it into an electrical signal that can be read by Arduino. Various sensors work differently, so choosing the right one is crucial.
Types of Temperature Sensors Compatible with Arduino
Sensor | Type | Accuracy | Best for |
---|---|---|---|
LM35 | Analog | ±0.5°C | Basic projects |
DHT11 | Digital | ±2°C | Humidity + Temp |
DHT22 | Digital | ±0.5°C | More precise than DHT11 |
TMP36 | Analog | ±0.5°C | Low power applications |
DS18B20 | Digital | ±0.5°C | Waterproof applications |
GY-906 | IR | ±0.5°C | Non-contact temperature measurement |
Choosing the Right Sensor
If you need basic temperature readings, LM35 is a great choice. For humidity + temperature, go with DHT11 or DHT22. DS18B20 is ideal for water temperature monitoring, while GY-906 is perfect for contactless measurement.
Required Components
- Arduino Uno
- Temperature sensor (e.g., LM35, DHT11, etc.)
- Jumper wires
- Breadboard
- 16×2 LCD display (optional, for output display)
Circuit Diagram & Connections

LM35 Wiring:
- VCC – Connect to 5V on Arduino
- GND – Connect to GND
- OUT – Connect to A0 (Analog pin)
DHT11 Wiring:
- VCC – Connect to 5V on Arduino
- GND – Connect to GND
- DATA – Connect to Digital Pin 2
Arduino Code for Temperature Measurement
void setup() {
Serial.begin(9600);
}
void loop() {
int sensorValue = analogRead(A0);
float temperature = (sensorValue * 5.0 * 100.0) / 1024;
Serial.print(“Temperature: “);
Serial.print(temperature);
Serial.println(” °C”);
delay(1000);
}
Displaying Temperature on an LCD
- If you want to display the temperature on an LCD, connect a 16×2 LCD screen and use the LiquidCrystal library.
Enhancing Your Project
- Data Logging: Store readings on an SD card.
- IoT Integration: Send data to a web dashboard using ESP8266.
- Temperature Alerts: Activate an alarm when a threshold is exceeded.
Common Issues & Troubleshooting
- Incorrect Readings? Check wiring and ensure the sensor is powered correctly.
- Fluctuating Readings? Add a capacitor across VCC and GND.
- Sensor Not Responding? Confirm the correct pin connection and software libraries.
Conclusion
Now that you’ve successfully built a temperature measurement system with Arduino, experiment with different sensors and enhance your project. Have questions? Drop them in the comments!
Additionally, for those who want to take things further, check out how to control Arduino via Android Bluetooth.
FAQs
What is the best temperature sensor for Arduino?
LM35 for basic projects, DS18B20 for waterproof applications, and GY-906 for contactless readings.
How do I connect an LM35 sensor to Arduino?
Connect VCC to 5V, GND to GND, and OUT to A0.
Can I use multiple sensors with Arduino?
Yes! Use multiple digital or analog pins to connect multiple sensors.
How do I display temperature on an LCD with Arduino?
Use a 16×2 LCD and the LiquidCrystal library.